How to access GPS location in Android phones program code
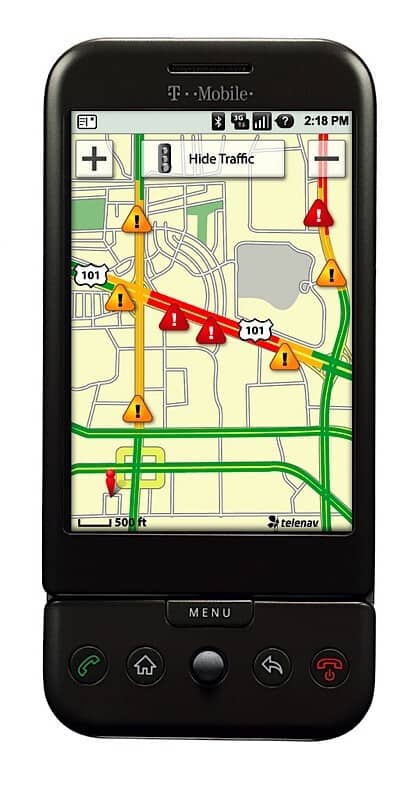
Following is a code for accessing the gps location in an Android phone by coding.Import necessary package to run it out.
package andgps.g;// ur package name
import com.ieffects.android.lbs.R;
import android.app.Activity;
import android.content.Context;
import android.location.Location;
import android.location.LocationListener;
import android.location.LocationManager;
import android.os.Bundle;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.Toast;
public class gps extends Activity {
private static final long MINIMUM_DISTANCE_CHANGE_FOR_UPDATES = 1; // in Meters
private static final long MINIMUM_TIME_BETWEEN_UPDATES = 1000; // in Milliseconds
protected LocationManager locationManager;
protected Button retrieveLocationButton;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
retrieveLocationButton = (Button)findViewById(R.id.retrieve_location_button);
locationManager = (LocationManager) getSystemService(Context.LOCATION_SERVICE);
locationManager.requestLocationUpdates(
LocationManager.GPS_PROVIDER,
MINIMUM_TIME_BETWEEN_UPDATES,
MINIMUM_DISTANCE_CHANGE_FOR_UPDATES,
new MyLocationListener()
);
retrieveLocationButton.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
showCurrentLocation();
}
});
}
protected void showCurrentLocation() {
Location location = locationManager.getLastKnownLocation(LocationManager.GPS_PROVIDER);
if (location != null) {
String message = String.format(
"Current Location \n Longitude: %1$s \n Latitude: %2$s",
location.getLongitude(), location.getLatitude()
);
Toast.makeText(gps.this, message,
Toast.LENGTH_LONG).show();
}
if (location == null)
{
Toast.makeText(gps.this, "No Location Available ", Toast.LENGTH_LONG).show();
}
}
private class MyLocationListener implements LocationListener {
public void onLocationChanged(Location location) {
String message = String.format(
"New Location \n Longitude: %1$s \n Latitude: %2$s",
location.getLongitude(), location.getLatitude()
);
Toast.makeText(gps.this, message, Toast.LENGTH_LONG).show();
}
public void onStatusChanged(String s, int i, Bundle b) {
Toast.makeText(gps.this, "Provider status changed",
Toast.LENGTH_LONG).show();
}
public void onProviderDisabled(String s) {
Toast.makeText(gps.this,
"Provider disabled by the user. GPS turned off",
Toast.LENGTH_LONG).show();
}
public void onProviderEnabled(String s) {
Toast.makeText(gps.this,
"Provider enabled by the user. GPS turned on",
Toast.LENGTH_LONG).show();
}
}
}
how do you set a location for the emulator?
ReplyDeletethere is an option in the emulator .. in thnk its in tools.
ReplyDeleteI'm going through your post, I seem to be missing the import com.ieffects.android.lbs.R library in eclipse. How do I get it exactly? I'm new to Java and eclipse
ReplyDelete